Often, there is the need of generating a random RGB color for LEDs. The most common approach is to obtain a random value between 0 and 1 (or 0 and 255) for the Red, Green and Blue channels.
RGB cube
This approach, however, leads to dark and light colors which may not be goal when picking a random color for a RGB LED, we want color!
As such, an alternative is to pick a color in the HSV space, more specifically in the circle at the top of the HSV cone (value=1
). While this avoids dark colors, there is a large probability of getting light (pastel) colors. Thus the search for random colors can be constrained to be nearby the rim of such circle, this is to say saturation cannot be smaller than 0.8.
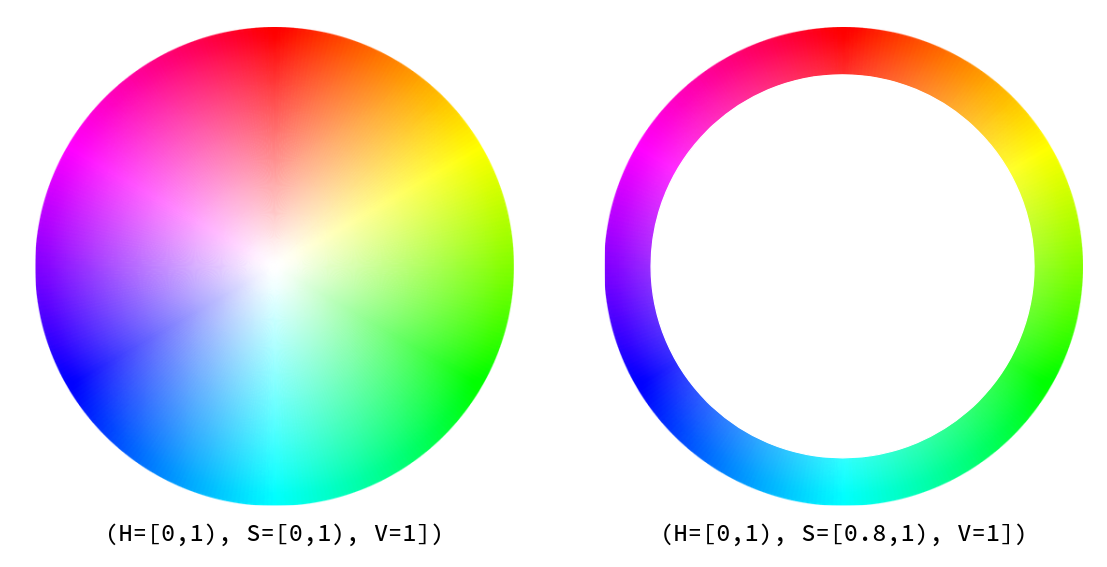
Circle at the top of the HSV cone (
value=1
). Left, saturation=[0, 1)
. Right, saturation=[0.8, 1)
.
Lastly, this Python scripts shows the results of picking 625 colors from the RGB space (left) and the outer rim of the value=1
HSV circle (right).
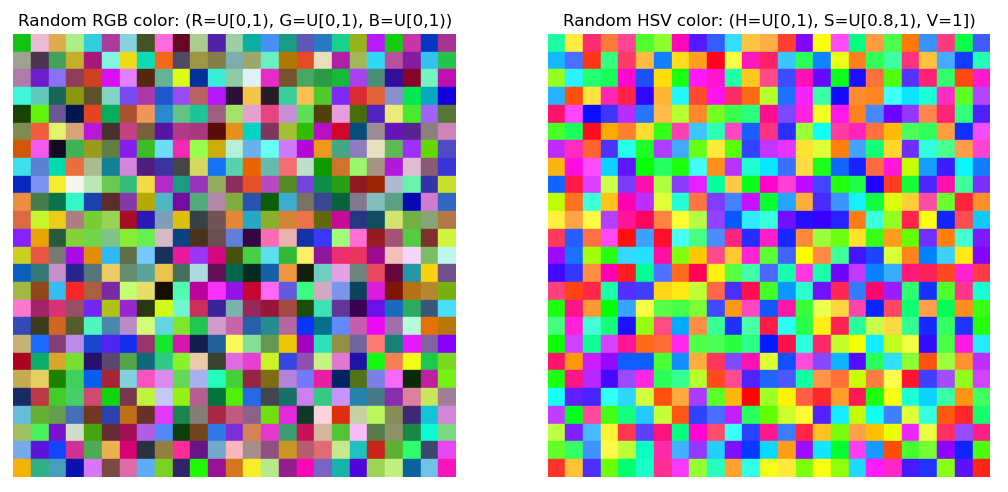
Picking random colors from the RGB space (left) and the outer rim of top of the HSV cone (right).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 |
|
Comments
comments powered by Disqus